reference:
- * jenkinsci/jenkins/jenkinsfile | * jenkins-infra/pipeline-library
- Pipeline Examples
- Jenkins Pipeline Syantx
- Jenkins Pipeline Cookbook
- Pipeline Steps Reference
- Pipeline Utility Steps
- Jenkins Pipeline Cookbook
- * declarative-pipeline-examples
- Class Exception
- Understanding the Differences Between Jenkins Scripted and Declarative Pipeline: A Comprehensive Guide with Real-World Examples
[!NOTE|label:good examples for pipeline flow:]
jenkins API
update node name
get label
@NonCPS def getLabel(){ for (node in jenkins.model.Jenkins.instance.nodes) { if (node.getNodeName().toString().equals("${env.NODE_NAME}".toString())) { currentLabel = node.getLabelString() return currentLabel } } }
set label
@NonCPS def updateLabel(nodeName, newLabel) { def node = jenkins.model.Jenkins.instance.getNode(nodeName) if (node) { node.setLabelString(newLabel) node.save() } }
Jenkinsfile Example
String curLabel = null String newLabel = null String testNodeName = null String curProject = env.JOB_NAME String curBuildNumber = env.BUILD_NUMBER node( 'controller' ) { try{ stage("reserve node") { node("${params.tmNode}") { testNodeName = env.NODE_NAME curLabel = getLabel() newLabel = "${curLabel}~running_${curProject}#${curBuildNumber}" echo "~~> lock ${testNodeName}. update lable: ${curLabel} ~> ${newLabel}" updateLabel(testNodeName, newLabel) } // node } // reserve stage } finally { if (newLabel) { stage("release node") { nodeLabels = "${newLabel}".split('~') orgLabel = nodeLabels[0] echo "~~> release ${testNodeName}. update lable ${newLabel} ~> ${orgLabel}" updateLabel(testNodeName, orgLabel) } // release stage } // if } // finally
raw build
reference:
Map buildResult = [:]
node( 'controller' ) {
buildResult = build job: '/marslo/artifactory-lib',
propagate: true,
wait: true
buildResult.each { println it }
println """
"result" : ${buildResult.result}
"getBuildVariables()" : ${buildResult.getBuildVariables()}
"getBuildVariables().mytest" : ${buildResult.getBuildVariables().mytest}
"getRawBuild().getEnvVars()" : ${buildResult.getRawBuild().getEnvVars()}
"getRawBuild().getEnvironment()" : ${buildResult.getRawBuild.getEnvironment()}
"getBuildCauses()" : ${buildResult.getBuildCauses()}
"getChangeSets()" : ${buildResult.getChangeSets()}
"buildVariables["mytest"]" : ${buildResult.buildVariables["mytest"]}
"buildResult.rawBuild" : ${buildResult.rawBuild}
"buildResult.rawBuild.log" : ${buildResult.rawBuild.log}
"rawBuild.environment.RUN_CHANGES_DISPLAY_URL" : ${buildResult.rawBuild.environment.RUN_CHANGES_DISPLAY_URL}
"""
} // node
get changeSets
reference:
def res = [:]
timestamps { ansiColor('xterm') {
node( 'controller' ){
cleanWs()
buildResult = build '/marslo/down'
if( currentBuild.rawBuild.changeSets.isEmpty() ) {
println "no new code committed"
} else {
buildResult.rawBuild.changeSets.each { cs ->
cs.items.each { item ->
println """
-----------------------------
revision : ${item.commitId}
author : ${item.author}
message : ${item.msg}
affected files :
\t\t${item.affectedFiles.collect{ f -> f.editType.name + ' - ' + f.path }.join('\n\t\t\t\t')}
"""
}
}
}
}
}}
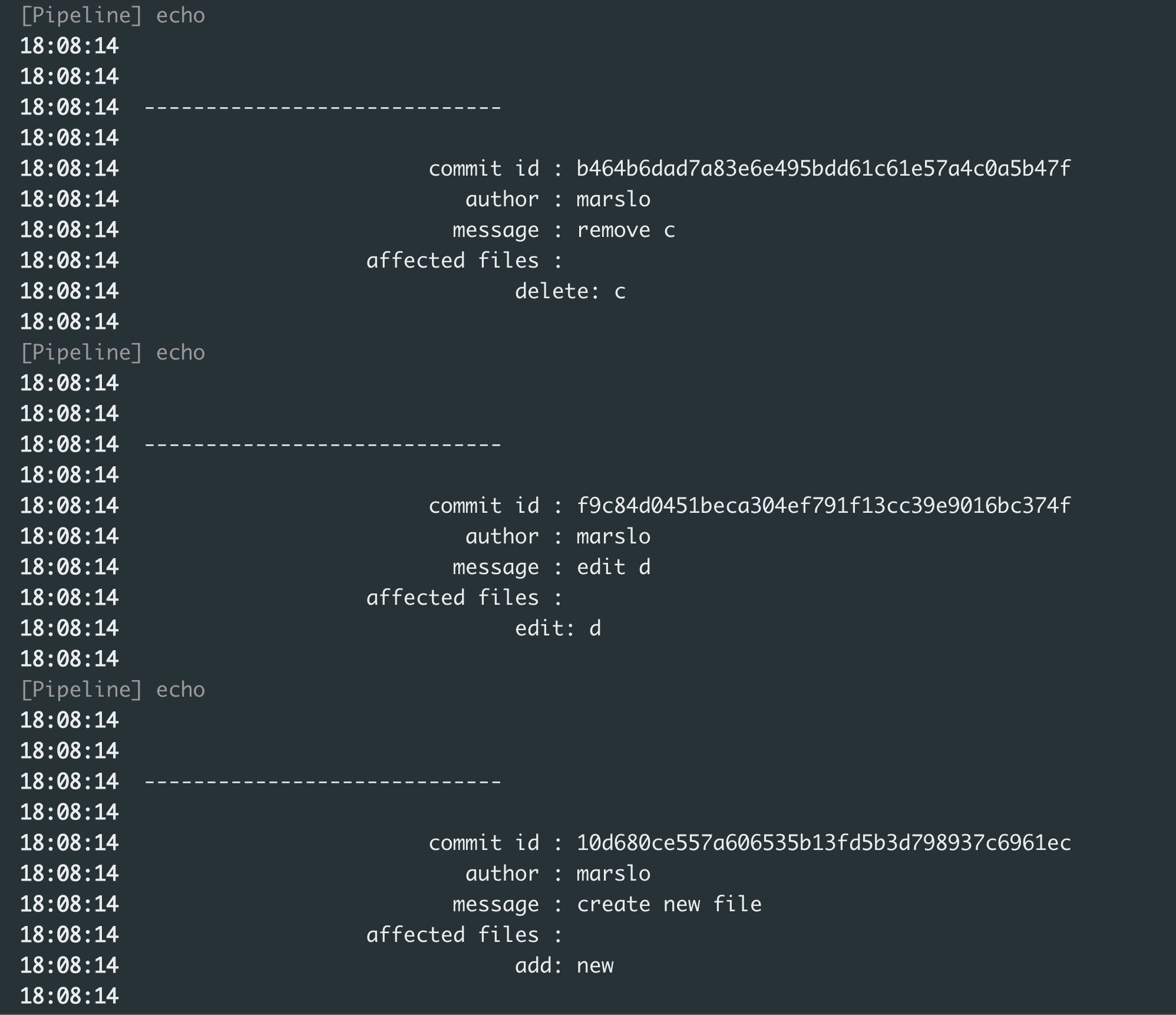
via api
$ curl -sSg \ https://jenkins.sample.com/job/<group>/job/<pipeline>/<buildId>/api/json | jq -r .changeSets[] # or $ curl -sSg \ https://jenkins.sample.com/job/<group>/job/<pipeline>/<buildId>/api/json?depth=100&&tree=changeSets[*[*]]
manager.build.result.isBetterThan
if( manager.build.result.isBetterThan(hudson.model.Result.UNSTABLE) ) {
def cmd = 'ssh -p 29418 $host gerrit review --verified +1 --code --review +2 --submit $GERRIT_CHANGE_NUMBER,$GERRIT_PATCHSET_NUMBER'
cmd = manager.build.environment.expand(cmd)
manager.listener.logger.println("Merge review: '$cmd'")
def p = "$cmd".execute()
manager.listener.logger.println(p.in.text)
manager.addShortText("M")
}
customized build
display name
currentBuild.displayName = '#' + Integer.toString(currentBuild.number) + ' mytest'
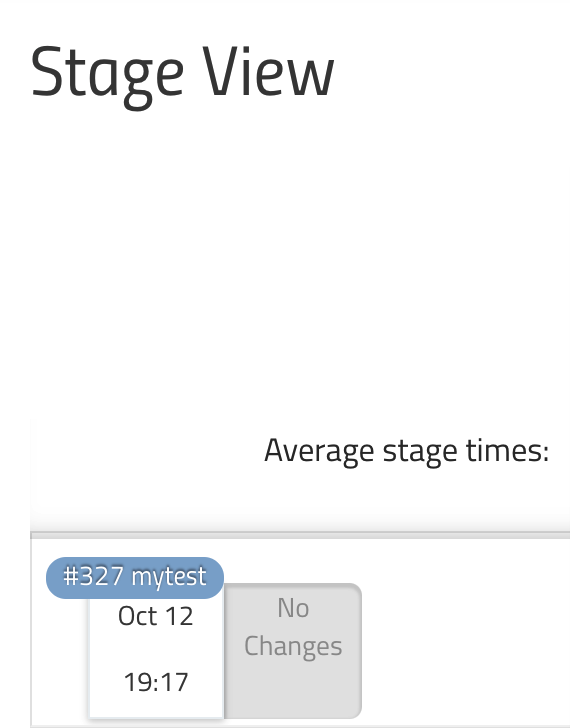
description
currentBuild.description = 'this is whitebox'
exception
[!TIP|label:references:]
using hudson.AbortException
import hudson.AbortException
throw new AbortException( "throw aborted exception" )
show catch message
try {
throw new AbortException( "throw aborted exception" )
} catch(e) {
def sw = new StringWriter()
e.printStackTrace( new PrintWriter(sw) )
println sw.toString()
// throw e // if not throw error, the catch process will only print the error message
}
withCredentials
import com.cloudbees.plugins.credentials.CredentialsProvider
import com.cloudbees.plugins.credentials.common.StandardCredentials
def sample( String credential ) {
[
inSSH : { println ".. ${credential}: ssh credential" } ,
inHttps : { println ".. ${credential}: git access token" }
]
}
def runWithCredentials ( String credential ) {
Closure classifyCredential = { cid ->
[
( com.cloudbees.plugins.credentials.impl.UsernamePasswordCredentialsImpl ) : {
sample( credential ).inHttps()
} ,
( com.cloudbees.jenkins.plugins.sshcredentials.impl.BasicSSHUserPrivateKey ) : {
sample( credential ).inSSH()
}
].find { it.key.isInstance(cid) }.value.call()
}
classifyCredential CredentialsProvider.lookupCredentials( StandardCredentials.class, jenkins.model.Jenkins.instance ).find { credential == it.id }
}
[ 'GITHUB_ACCESS_TOKEN', 'GITHUB_SSH_CREDENTIAL' ].each { runWithCredentials(it) }
- result
.. GITHUB_ACCESS_TOKEN: git access token .. GITHUB_SSH_CREDENTIAL: ssh credneital